Category Archives: NSS
Simple SHA256 Hashing with NSS in C
Here we present a program that calculates SHA256 of the string "abc"
as a demonstration of how to use Mozilla’s Network Security Services library. We assume the reader has compiled a working library, or installed one through a package manager. The build instruction in this tutorial assume Windows and Visual Studio 2019 with clang
.
My comments are overly verbose, and this should make it easier for beginners to follow along.
Continue reading Simple SHA256 Hashing with NSS in C
Building Mozilla NSS on Windows 10
If you’re like me you may have searched the web on how to build the Mozilla Network Security Services on Windows 10. You may have found this obsolete project on Github. You may also have found the obsolete directions for Pidgin too.
On Windows the NSS build requriements are the same as for Firefox, so go look through the build instructions for Firefox on Windows. Install Visual Studio 2019 as directed, or add the packages you need with the Visual Studio Installer. And install the Mozilla Build package in the default directory.
Now that’s done, we have one more requirement left, and that’s Gyp. For Gyp, we first need Git. If you don’t have it already, install it now. At this point I’m not certain Git comes with Visual Studio 2019. It could be, but I have a separate install anyway.
All the instructions now assume you have opened an x64 Native Tools Command Prompt for VS 2019, so open one if you don’t have already.
I prefer to keep my software I build in C:\build
, so that’s what the directions will indicate.
cd c:\build git clone https://chromium.googlesource.com/external/gyp
Gyp is a Python program, so if you don’t have it already, add Python to your path. You’ll find it in C:\mozilla-build\python
and also add gyp to your path, like so
set path=%path%;C:\mozilla-build\python;C:\build\gyp
Download and extract nss-3.45-with-nspr-4.21.tar.gz By the time you read this, that particular download might be obsolete, so please adjust as needed.
I use 7z for my command line examples.
cd c:\build 7z x %homepath%\Downloads\nss-3.45-with-nspr-4.21.tar.gz
That only creates the tar file, so we need to run 7z again.
7z x nss-3.45-with-nspr-4.21.tar
You may need to add vswhere
to your path, and you can do that now with
set path=%path%;%ProgramFiles(x86)%\Microsoft Visual Studio\Installer
At this point we’ll need an msys shell. So still in your x64 Native Tools Command Prompt for VS 2019,
cd C:\mozilla-build\msys msys
In msys, cd to the place where you extracted NSS.
cd /c/build/nss-3.45/nss
And build with
./build.sh
And you should get a nicely compiled debug build in C:\build\nss-3.45\dist
. Happy hacking with NSS.
Contact
The author can be reached at johann@myrkraverk.com.
The Case of the Apparent NSS Memory Corruption
This is a story of my encounter with an apparent memory corruption issue in the Netscape Security Services library.
The source I’m discussing can be found on Github.
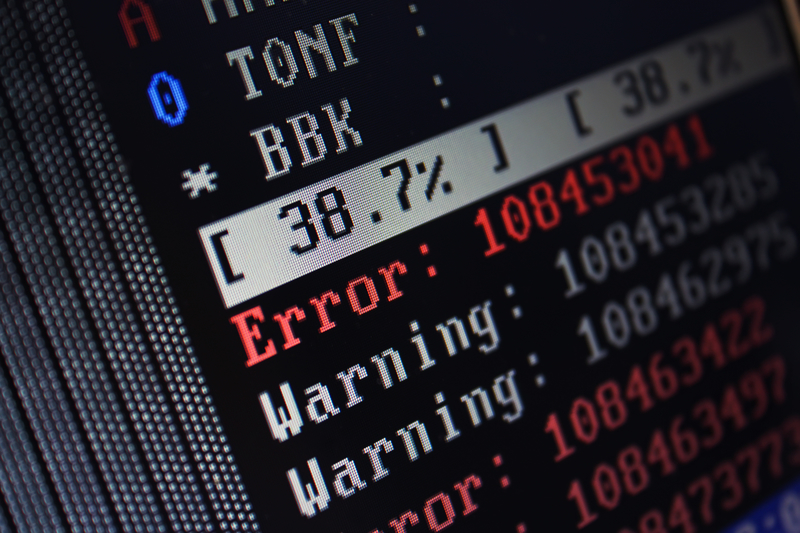
Usually, when I try to get acquainted with a new API, I start to write simple program, one API call by call, which I compile and run after each step.
Imagine my surprise, when after adding the following function call (the only thing I added)
PK11_FindKeyByAnyCert( certificate, passwd );
I got this memory corruption error.
dblfree(56630,0x7fff73f61300) malloc: *** error for object 0x7fd39250ce70: pointer being freed was not allocated *** set a breakpoint in malloc_error_break to debug zsh: abort ./dblfree
The above error is taken from my minimal example of the problem, not the actual program I was working on at the time. The only difference is the name of the binary and the hex numbers.
So what is happening here? I didn’t know. And to find out, it’s really important to use the right tool for the job.
So the first thing I did was to instrument my code with the built-in OS X tools, instruments(1). That didn’t tell me much; either because it doesn’t help in this particular instance, or that I just don’t know how to use it.
I will make a note that some people suggested Valgrind. I didn’t go that way because the problem seems to be adequately described with the Clang Address Sanitizer.
Continue reading The Case of the Apparent NSS Memory Corruption